What would you expect the following piece of Code to print. if the directory 'A' doesn't exist
@ECHO OFF
IF '1'=='1' (
CD a
ECHO %ERRORLEVEL%
)
CD a
ECHO %ERRORLEVEL%
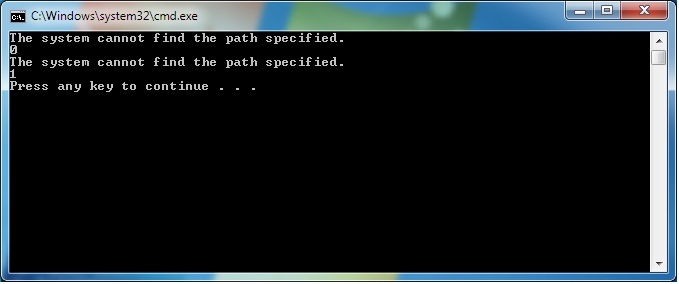
Not very intuitive right?
- SET ENABLEDELAYEDEXPANSION at the top of your script.
- Replace % delimited variables with an Exclamation. i.e. %ERRORLEVEL% becomes !ERRORLEVEL!
Now our script looks like this, and behaves as expected.
Working Script
@ECHO OFF
REM Enable Delayed Expansion
setlocal enabledelayedexpansion
IF '1'=='1' (
CD a
REM Use Exclamations instead of percentages
ECHO !ERRORLEVEL!
)
CD a
ECHO %ERRORLEVEL%
~Eoin Campbell